概要
serviceを作成しての環境変数の扱いかたについて説明したいと思います。
コード
.envの内容以下にする
LISTEN_PORT=3000
DB_HOST=localhost
DB_PORT=3306
DB_USERNAME=testuser
DB_PASSWORD=testpasswd
DB_DATABASE=testdatabase
モジュールインストール
$ npm install @nestjs/config
serviceソース
$ vi ./src/config.service.ts
import { Injectable } from '@nestjs/common';
import { ConfigService as NestConfigService } from '@nestjs/config';
@Injectable()
export class ConfigService {
get listenPort(): string {
return this.configService.get<string>('LISTEN_PORT', '3000');
}
get dbHost(): string {
return this.configService.get<string>('DB_HOST', 'localhost');
}
...
...
}
ConfigService
がAppModule
のプロバイダとして追加されると、NestJSはアプリケーションの起動時にConfigService
のインスタンスを作成します。
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { ConfigService } from './config.service';
@Module({
imports: [ConfigModule.forRoot({
isGlobal: true
})],
controllers: [AppController],
providers: [AppService, ConfigService],
exports: [ConfigService],
})
export class AppModule {}
main.ts
でConfigService
を直接使用することは一般的なパターンではありませんが、NestJSの柔軟性により、特定のシナリオでこれを実現することは可能です。ConfigService
からポート番号を取得してアプリケーションを起動してみたいと思います。
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import { ConfigService } from './config.service';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
const config = app.get(ConfigService);
await app.listen(config.listenPort || 3000);
}
bootstrap();
起動してみます。
$ npm start
> testdotenv@0.0.1 start
> nest start
[Nest] 9616 - ... LOG [NestFactory] Starting Nest application...
[Nest] 9616 - ... LOG [InstanceLoader] AppModule dependencies initialized +10ms
[Nest] 9616 - ... LOG [RoutesResolver] AppController {/}: +9ms
[Nest] 9616 - ... LOG [RouterExplorer] Mapped {/, GET} route +3ms
[Nest] 9616 - ... LOG [NestApplication] Nest application successfully started +2ms
APIの呼び出しで確認します。簡単にブラウザからも可能です。
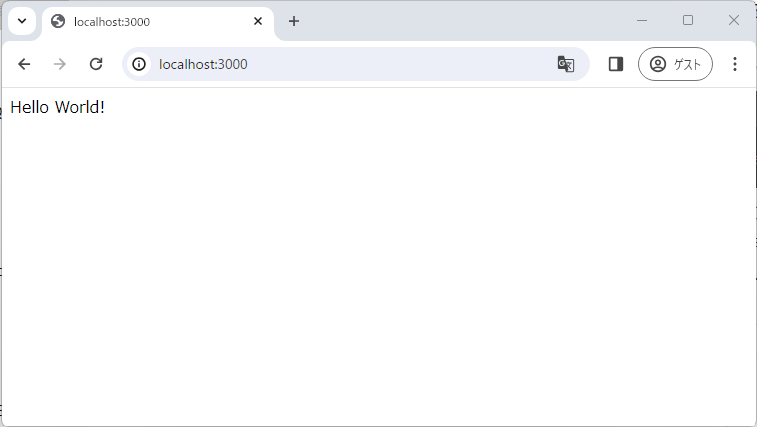