概要
Express.jsとTypeScriptを使用してプロジェクトを開始する方法についての記述したいと思います。ここでは、プロジェクトのセットアップから、環境変数の設定、そして基本的なCRUD操作の実装までをカバーします。
初期設定
新しいフォルダーを作成して基本的な設定をしたいとおもいます。
$ mkdir express-pj
$ cd express-pj
$ npm init -y
Wrote to ... ... ...
{
"name": "express-pj",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
必要なパッケージをインストールします。expres, typescript周りのモジュール。
$ npm install express dotenv
$ npm install -D typescript ts-node @types/node @types/express
Typescript関連設定のtsconfig.json、ほとんどはデフォルトで良いですがoutDirは修正をお勧めします。
...
"outDir": "./dist",
...
環境変数ファイル「.env」を用意します
PORT=[このサーバーの接続ポート] ← 例) 3000
DB_HOST=[ホスト名] ← 例) localhost
DB_USER=[データーベースユーザーID] ← 例) testuser
DB_PASS=[データーベースユーザー暗証番号] ← 例) testpasswd
DB_NAME=[データーベース名] ← 例) testdb
コードを作成
index.tsを作成
srcフォルダーを作成しその中にindex.tsを作成します。
import express from 'express';
const app = express();
const port = 3000;
app.listen(3000, () => {
console.log(`Server is running on port:${port}`);
});
実行してみます。
$ npx ts-node src/index.ts
Server is running on port:3000
APIを追加
環境変数の読み取り
import dotenv from 'dotenv';
dotenv.config();
const app = express();
const port = process.env.PORT || 3000;
jsonミドルウェア
app.use(express.json());
ルーティングを追加
app.get('/users', (req, res) => {
res.json(users);
});
app.post('/users', (req, res) => {
const newUser = { id: users.length + 1, ...req.body };
users.push(newUser);
res.status(201).json(newUser);
});
app.put('/users/:id', (req, res) => {
const { id } = req.params;
const index = users.findIndex(user => user.id === parseInt(id));
if (index === -1) {
return res.status(404).json({ message: 'User not found' });
}
users[index] = { ...users[index], ...req.body };
res.json(users[index]);
});
データー
固定テータを作成して実行してみます
const users = [
{ id: 0, name: 'J.Taro', age: 35 },
{ id: 1, name: 'S.Hanako', age: 53 },
{ id: 2, name: 'K.Jiro', age: 45 }
];
実行してみる
$ npx ts-node src/index.ts
Server is running on http://localhost:3000
ブラウザで確認してみる
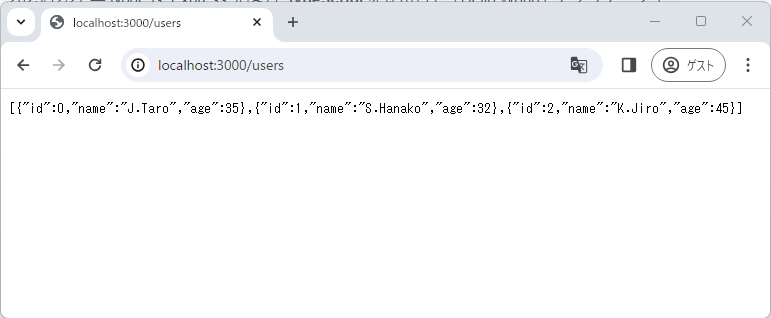